Have you ever encountered the frustrating error message “could not convert string to float” while working with numerical data in Python? If you have, you’re not alone. In this article, I’ll delve into the reasons behind this error and provide you with practical solutions to overcome it.
When working with numerical data in Python, it’s common to come across situations where you need to convert a string to a float. However, sometimes the conversion process can throw an error, leaving you scratching your head. But fear not! I’ll guide you through the common causes of this error and show you how to fix it, so you can get back to analyzing your data without any hiccups.
Could Not Convert String to Float
Understanding the error message
When working with numerical data in Python, it is common to encounter the error message “could not convert string to float.” This error message occurs when there is a problem converting a string value to a float data type.
The “could not convert string to float” error message is a type of ValueError that Python raises when it tries to convert a string to a floating-point number but encounters an issue. This error typically occurs when you are attempting to convert a string to a float using the float() function or when performing arithmetic operations that involve string inputs.
Causes of the error
There can be several causes for the “could not convert string to float” error. Some of the common causes include:
- Formatting issues: The string might contain characters or symbols that cannot be converted to a float, such as commas, dollar signs, or spaces. It is important to ensure that the string is properly formatted before attempting the conversion.
- Missing or incorrect decimal point: If the string represents a decimal number, it must contain a decimal point. If the decimal point is missing or placed incorrectly, the conversion will fail.
- Non-numeric characters: The string may contain non-numeric characters that cannot be converted to a float. This can include alphabetic characters, special symbols, or any non-numeric characters.
- Input validation: If the string input is not properly validated, it could include unexpected characters or formatting that cannot be converted to a float. It is important to validate and sanitize the input data to avoid such errors.
Overall, understanding the “could not convert string to float” error message and its causes is essential in troubleshooting and fixing this issue in Python. By addressing the specific cause of the error, you can ensure smooth data analysis and avoid interruptions in your programming workflow.
How to Fix the “Could Not Convert String to Float” Error
Check for non-numeric characters: One of the common causes of the “could not convert string to float” error is the presence of non-numeric characters in the string. To fix this error, you can check for any non-numeric characters in the string before converting it to a float. Use the isnumeric() method to determine if the string contains only numeric characters. If it doesn’t, you can remove or replace the non-numeric characters before converting the string to a float.
Convert string to float using the float() function: Another way to fix the “could not convert string to float” error is to use the float() function in Python. The float() function takes a string as input and returns its floating-point representation. When using the float() function, make sure that the string you pass as an argument is in a valid format for a float, with no non-numeric characters and the decimal point in the correct position.
Handle empty or missing values: Sometimes, the “could not convert string to float” error can occur if the string is empty or has missing values. To fix this error, you can check if the string is empty or contains missing values before attempting to convert it to a float. If the string is empty or contains missing values, you can either skip the conversion or provide a default value to use instead.
Use exception handling to handle the error: Exception handling is a powerful technique that can be used to handle and recover from errors in Python. When encountering the “could not convert string to float” error, you can use a try-except block to catch the error and handle it gracefully. Inside the try block, you can attempt to convert the string to a float. If the conversion fails, the code inside the except block will be executed, allowing you to handle the error in a specific way, such as displaying an error message or taking alternative actions.
By following these steps, you can effectively fix the “could not convert string to float” error in Python. Remember to always validate and sanitize your input data to prevent errors and ensure smooth execution of your code.
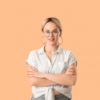
Jessica has a flair for writing engaging blogs and articles. She enjoys reading and learning new things which enables her to write different topics and fields with ease. She also strives to break down complex concepts and make them easy for anybody to comprehend.